Beginners in Java — Getting Started with OOP
Java is an object oriented programming language — what does that mean?
Whenever a programming methodology is based on objects or classes, instead of just functions and procedures it is called Object Oriented Programming (OOP).
All code in Java must be contained within classes
Objects in an object oriented language are organised into classes or in simpler terms a class gives birth to objects. Java is one such programming language.
Classes and Objects
Everything in a java program is contained within a class. A class can be considered as a factory that produces objects. An object contains data inside of it. It can have one data item or many. e.g. a lunchbox object might contain 3 data items
- Fries (of the type solid food)
- Burger (of the type solid food)
- Cold Drink (of the type liquid food)
These 3 different items inside our object are called fields. Every field must have a type. In the above example, we have 3 fields but 2 of them have the same type. At the same time we methods that act on data. For example, methods can be used to see what data is contained inside an object. These are called accessor methods. We can also have methods that modify the data inside the object. These are known as updater methods.
Let’s say that we have a food factory (class). It makes lunch boxes (objects). A lunchbox contains 3 different items of food(fields). Two of them are the same type (solid food) and one is different (liquid food). We have 3 people who interact to use this food. The chef (Method 1) heats the food, the waiter (Method 2) serves the food and the consumer (Method 3) eats the food.
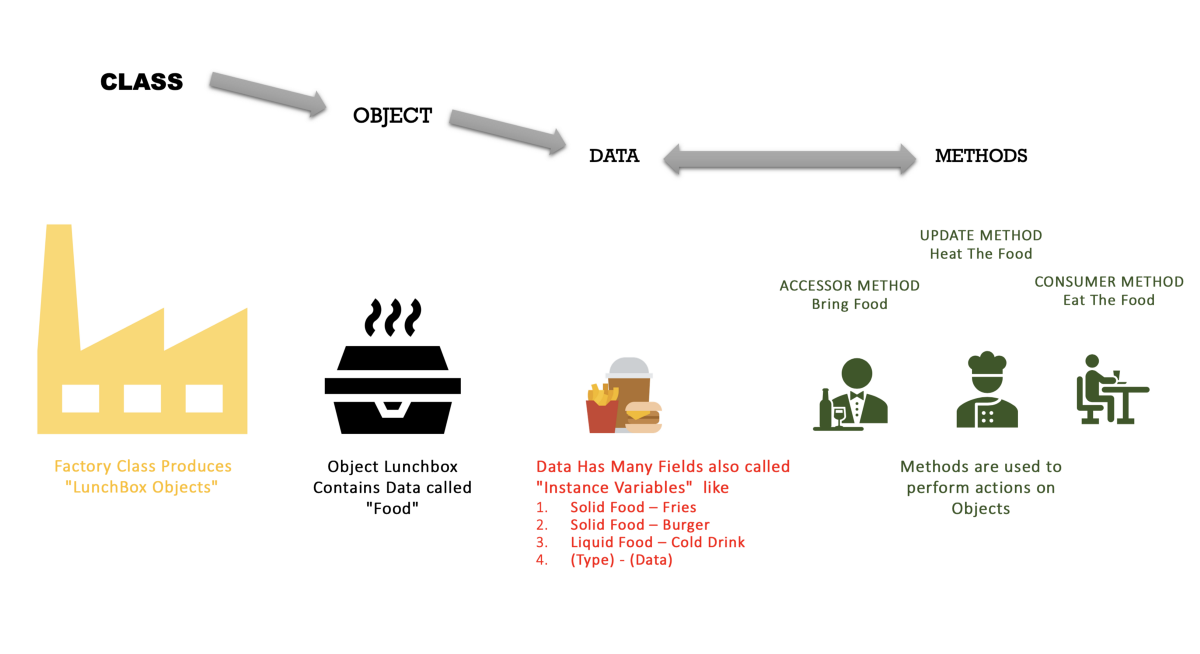
In Java programs, the primary “actors” are objects. Every object is an instance of a class, which serves as the type of the object and as a blueprint, defining the data which the object stores and the methods for accessing and modifying that data.
— Michael T. Goodrich, Roberto Tamassia, Michael H. Goldwasser (Data Structures and Algorithms in Java)
Understanding Objects
An object is an instance of the class i.e. A class can give rise to many objects. For example
Object 1
- Solid Food Item 1 — Fries
- Solid Food Item 2 — Burger
- Liquid Food Item 1 — Cold Drink
Object 2
- Solid Food Item 1 — Rice
- Solid Food Item 2 — Fish
- Liquid Food Item 1 — Water
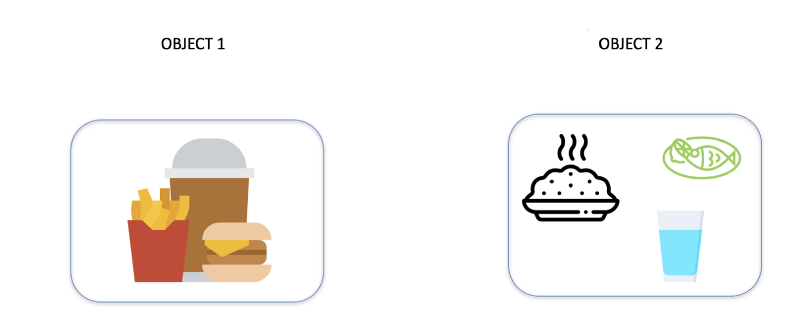
The Process
Let’s try to see how to build a basic Factory Class. Let’s first take a look at the complete code.
Filename
The lines public class Factory{} enclose the complete code. The file has to be named as the class name. So the filename is also Factory.java.
public class Factory{
...
}
Fields/Instance Variables
Now the top 3 lines give you the 3 items (instance variables) you want in your lunchbox. Right now you can see that all 3 have the same type = String. Java gives you the flexibility to create your own types which essentially means creating a class by that name. So if you were to create a SolidFood class, for example, you would have to write public class SolidFood{} and that’s all.
Right now we have 3 instance variables of the type String and names SolidFood1, SolidFood2 and LiquidFood1.
private String SolidFood1; // Solid Food 1st Instance Variable
private String SolidFood2; // Solid Food 2nd Instance Variable
private String LiquidFood1; // Liquid Food 3rd Instance Variable
Constructors
The code that creates an object lies inside the constructor. A constructor can be an empty one or can take some input arguments that are used to define the object. In our case sf1, sf2 and lf1 are the arguments that define what items are contained in our lunchbox.
In simple words, a constructor defines what the fields of the object look like.
public Factory(){} // 0-arg constructor (Not used)
// 3-arg constructor
public Factory(String sf1, String sf2, String lf1){
SolidFood1 = sf1;
SolidFood2 = sf2;
LiquidFood1 = lf1;
}
Methods
Methods are used to either fetch object fields or modify them. In our case, we have only written fields that fetch the item names.
public String getSF1(){return SolidFood1;} // Method to get the name of 1st Instance Variable
public String getSF2(){return SolidFood2;} // Method to get the name of 2nd Instance Variable
public String getLF1(){return LiquidFood1;} // Method to get the name of 3rd Instance Variable
The main method is the only method that needs to be in every program. It is the only thing that java compiler runs. From inside the main, you can create objects, use methods on these objects and print stuff.
Object Creation
Object is created by calling a constructor. Once an object is created you can use various methods at your disposal to understand or modify the object. Here we are just showing how to access field names.
Factory lunchBox = new Factory("s1","s2", "l1"); // Object Created Yay!!!
How to run
Just in case you do not have java visit this page.
Assuming you have java installed in your system you should be able to run the program using the following commands.
> javac Factory.java (This command compiles your code into Machine Readable Code)
> java Factory (Running the Program)
The output you see looks like this

There is a lot more involved in OOP. This was just the beginning. Later articles will include more involved concepts of object oriented programming.
Member discussion