How to Code Inheritance in Java — Beginner’s Tutorial in OOP
Object Oriented Programming
Let’s understand the concept of ‘inheritance’ in object oriented programming
Webster’s online dictionary defines inheritance as the acquisition of a possession, condition, or trait from past generations. In object oriented design inheritance has a similar (not exactly the same) connotation. Inheritance means
- Organising classes in a hierarchy
- Higher hierarchy inherits properties from lower hierarchy
- Clubbing similar things into the same class
- Classes go from general to specific as you go higher in hierarchy
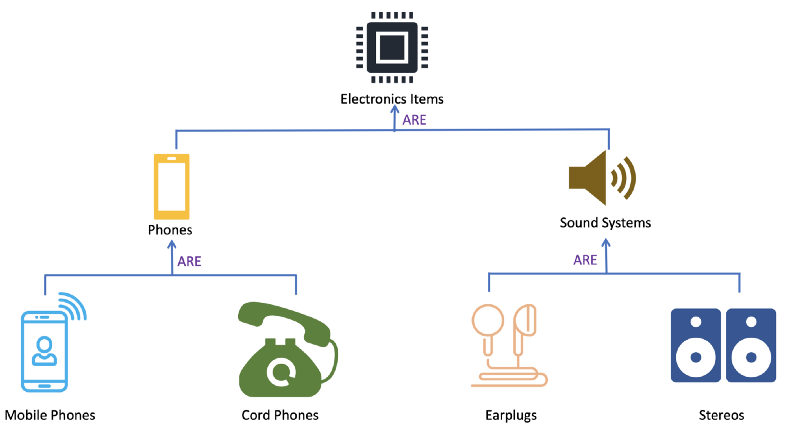
This means that the most basic class, also known as the base class, will be the most general one. It is also known as the parent class or the super-class. For example ‘electronics’ is the base class and its child class will be ‘mobile phones’ or ‘sound systems’.
To know more about the basics of what constructs a class, details about fields, methods and constructors, you can refer to this article.
Using mathematical notations, the set of phones is a subset of the set of electronics, but a superset of the set of landlines. The correspondence between levels is often referred to as an “is a” relationship, i.e. a phone is an electronics item, and a landline is a phone
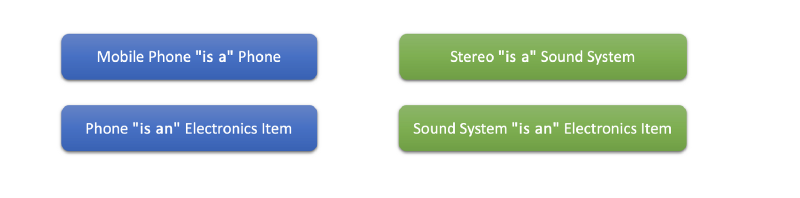
As we go higher in the hierarchy we become more and more specific.
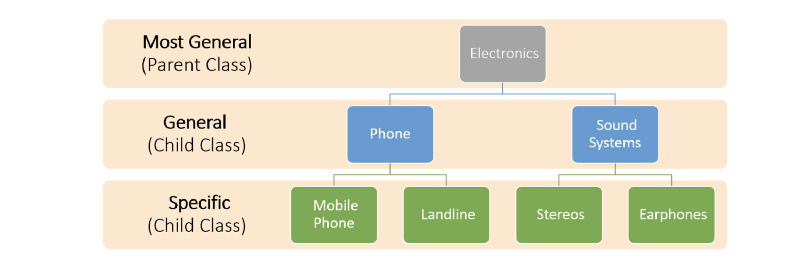
This hierarchical design is extremely useful because it promotes the reuse of code. The common functionality can be grouped together into the same class and differentiated behaviours can form the specific cases or the sub-classes.
In technical terms, we say that the subclass extends the superclass. When inheritance is used, the subclass automatically inherits all methods from the superclass (other than constructors). The subclass differentiates itself from its superclass by the following 2 methods.
- It can augment the superclass by adding new fields and new methods.
- It override existing behaviours by providing a new implementation of an existing method.
Class — A group of similar objects
Fields — Properties of a class
Objects — Instances of a class
Methods — Actions that can be applied on objects
Constructor — Action that is used to create an object
Enough theory. Let’s code!
As a simpler example let us consider the following hierarchy. Book as the base class and e-book and paper books as the 2 sub-classes.
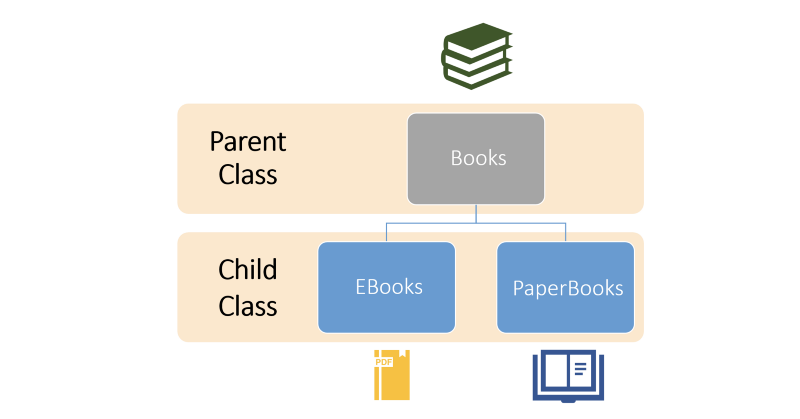
- Book fields — Author name, cost price, sale price, title and pages
- Book methods — netProfit() and getTax()
- EBook fields — downloadSite and sizeMB
- EBook methods — pageSize(), printTitle() and getTax()
Note that book fields are the properties that every book will necessarily have. Since e-book will inherit from the book class it will have the fields of the book class along with its own 2 fields.
Now the thing to notice is that not only can ebook access the 2 methods of the Book class, namely — netProfit() and getTax(), but it can also modify them. Note the modification of tax calculation in the code below. In the code below notice how EBook inherits from Book by using the keyword extends.
- Augmentation — EBook class augments Book class by adding the fields downloadSite and sizeMB and also by adding a method pageSize(). Note that downloadSite is something that is not relevant for PaperBooks so it cannot be put in the Book class. Also, pageSize() is measured in MBs hence it is specific to e-books.
- Override — e-books are charged an additional $2 tax apart from the 30% tax on the profits. Here we can see that EBook class overrides the original getTax() method by adding a fixed $2 charge on top of the original tax calculation.
We saw in the previous article, that it is the main method that actually runs when the program is run. Since we didn’t include any main method in the above 2 classes we will create a separate test file to write the main method and run these two classes.
The output of the testbook class clearly shows inheritance at play. We were able to print the fields of Book class even on an eBook.

Things to note
- Inheritance works by using the keywords extends
- Constructors are never inherited only the fields and the methods are
- Even if a class definition makes no explicit use of the extends clause, it automatically inherits from a class, java.lang.Object, which serves as the universal superclass in Java
- In Java, a constructor of the superclass is invoked by using the keyword super

The complete code is in the git repo mentioned below.
Member discussion