Javascript Basics — Var | Let | Const
A variable is a container for data. To use a variable we need to first declare it. To declare it we need some kind of an in-built statement. In javascript, we use three different types of statements to declare a variable.
- Var
- Let
- Const
Often people confuse these and end up using one when they need to use the other. Let’s quickly take a look at the difference between the workings of these statements.
Code Organization
Javascript can be executed in a number of ways. We will use the most popular way i.e. on a browser. Other ways include using Node.js or some C++ shell that extends V8 Engine.
We will create 2 files.
- Index.js — This will contain our actual javascript code.
- Index.html — This is the supporting file that contains an HTML button to execute index.js when we click the button. (We don’t need this file but why not code in style when we can!)
HTML Code
This just contains a heading and a button that runs the index.js file when clicked.
JS Code
Java script code will contain 4 functions
- varExample — A function to display var statement
- letExample — A function to display let statement
- constExample — A function to display const statement
- go — The function that will call each one of them when the button is clicked
The javascript code will look like this
function varExample() {
//var code goes here
}
function letExample() {
//let code goes here
}
function constExample() {
//const code goes here
}
function go() {
varExample();
letExample();
constExample();
}
Var Statement
The basic nature of var is such that when you declare a variable with it the variable’s scope exists beyond the block in which the variable is declared. Too much jargon?
In simpler words, if a variable for declared inside a for-loop, the variable should exist only inside the loop. But in the case of var statement the variable exists even after the loop ends. Let’s see an example.
The plain HTML page looks like this

Notice that the browser console is also open in this screenshot. We will see the output of our code in the console.
To open console in Google Chrome use the shortcut Option + ⌘ + J (on macOS), or Shift + CTRL + J (on Windows/Linux). The console will either open up within your existing Chrome window, or in a new window. You may have to select the Console tab
Let’s implement a simple for loop using var. Note the commented out console.log line. We will first run the code with it commented out and later we will uncomment this line and see what happens
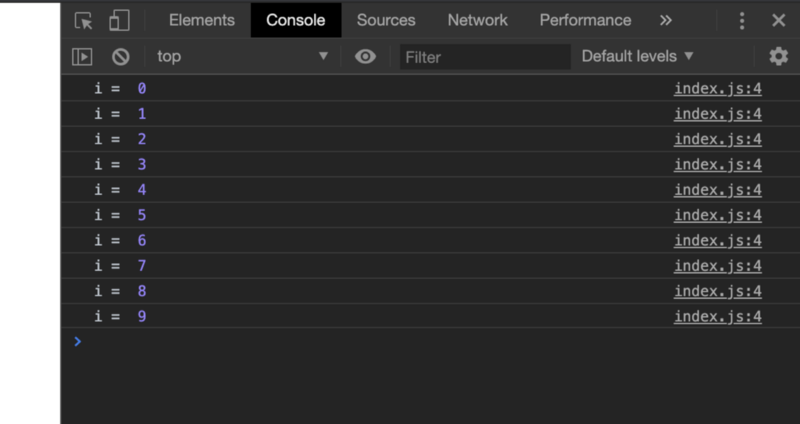
On clicking the Run JS Program button we get the following output in the console. Once i becomes equal to 10 it no longer enters the loop-block and thus doesn’t get printed. Now let’s uncomment the console.log line and see what happens.
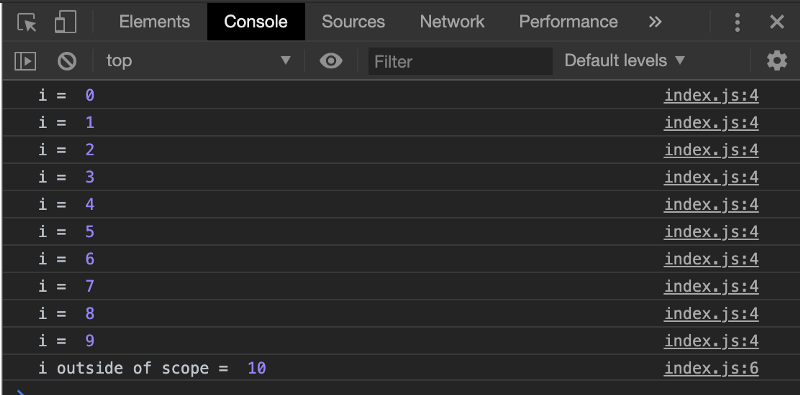
We can clearly see that when we use ‘var’ statement ‘i’ exists outside the loop as well.
Let Statement
The difference between Let Statement and Var Statement is that let exists only within the scope in which it is declared. Let’s re-run the same code as above by replacing var with let.
Let’s see what comes on the console.
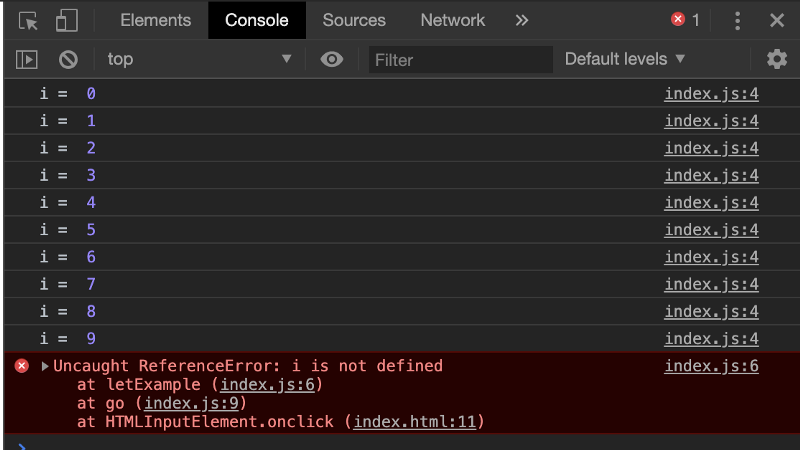
Voila! Javascript is unable to find ‘i’ this time around. Since let doesn’t allow the variable to exist outside the scope of the block, the js-engine throws an error.
Const Statement
Finally, the const statement is used to declare a variable that cannot change its value throughout the program. Such variables are used to hold information that you don’t want anyone to modify like global IPs, port numbers or other identifying information.
We declare k=1. Now when we try to change it to 2 the javascript engine will again throw an error.
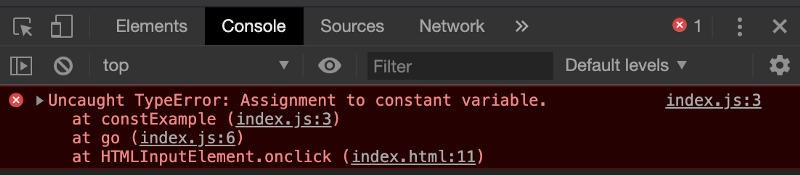
Assignment to constant variable throws TypeError.
Javascript is one of the most useful languages and its developers are the highest paid developers in the industry. It pays to know the basics of js before building huge projects with. This article can also be found on Medium.
Member discussion