Comprehensive Python Cheat Sheet for Beginners
16 minute one-stop tutorial for getting started with Python
Introduction
Python has rapidly become the most desired language in the job market. The wide applicability of Python, from web development to machine learning, is the reason why Python programmers are in a high demand. A simple comparative analysis with the other machine learning languages shows its increasing popularity on Google Trends.

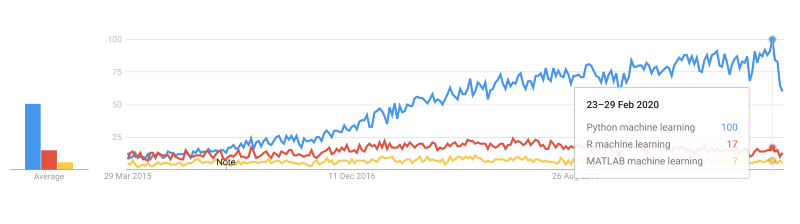
Python’s worldwide dominance is also shown by how frequently it is searched across the world.
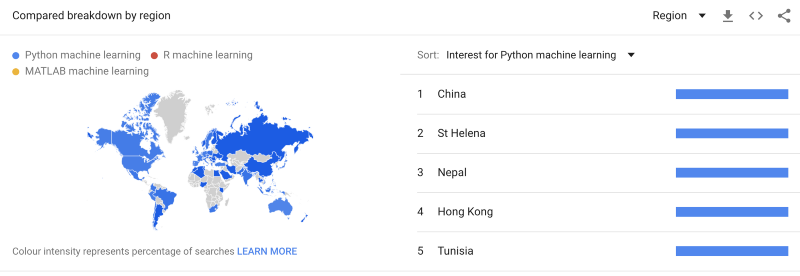
Python’s Community
The primary reason for Python’s growth is its open source development by a well-knit 30 year old community. Trends given by StackOverflow.com are a proof. See how Python has left behind three major programming languages — Java, C, C++.
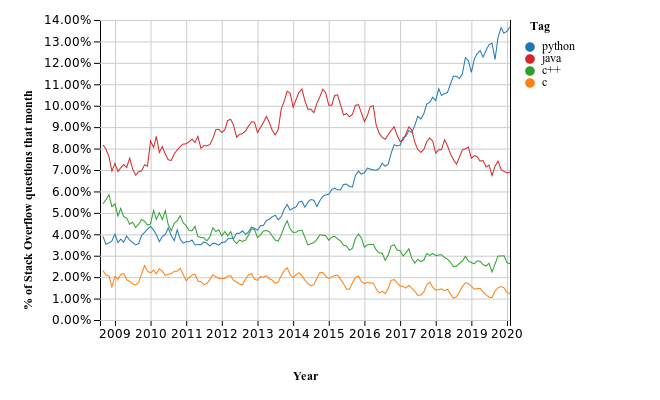
Even the corporate world loves Python. Google alone has created wildly popular machine learning libraries like Tensorflow, Keras etc and has distributed those for free.
Technically Speaking
Python is
- Interpreted
- Platform Independent
- Embeddable
- Dynamically typed
This means that Python is interpreted directly into machine understandable bytecode unlike C, C++ or Java which require separate compilation and execution steps. It is due to these properties that Python runs on everything from digital watches to Mars Rover.
In this cheat sheet we will be focusing on only Python 3 because on January 1st, 2020 the community decided to stop supporting Python 2 any further. This event was called Sunsetting Python 2.
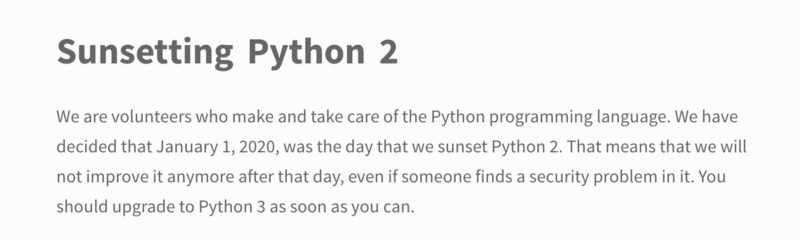
Types
The building block in Python is an object and objects have types. A type is a way of describing the data stored inside the object. For example, to store a number we have integer, float and complex types. The major categories of types in Python are boolean, numerics, sequences, mappings, classes and exceptions. We will cover all of them in details with examples but let us start with the basic types that are used most commonly.
Basic Types
Integers
In Python 3 the length of an integer is only limited by memory and nothing else. The type() function is used to get the type of the variable. Let’s see what this code prints on the terminal. The print function converts the binary, octal and hexadecimal forms to decimal before printing.
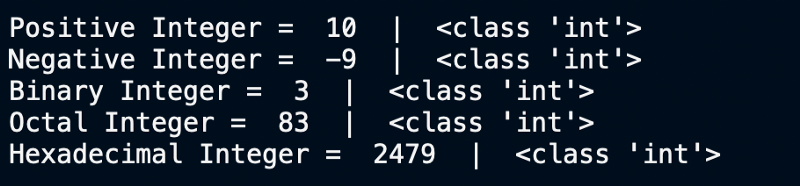
Complex, Float, Boolean, String and Byte
The other basic types are listed below
- The maximum value a float in Python can have is 1.8x10308. Anything bigger and Python labels it as inf i.e. infinity
- Complex numbers are specified as <real part> + <imaginary part>j
- Strings are sequences of characters. Strings are enclosed within a pair single or double quotes.
Let’s see when printed on the terminal how do they appear. Also, note the corresponding class (which is printed using the function type()).
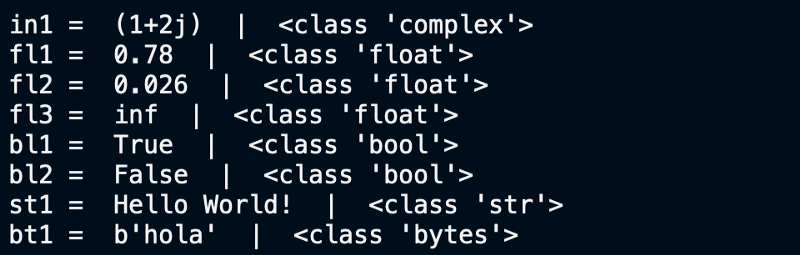
String
The string type is as vast as the ocean. One needs a separate section, if not an article to describe it.
Just like an integer a string can be as long as one wants. To check how long the string is we can use len() function. We will cover functions later but as of now consider it a block of code that perform a single task.
str1 = “I am a string”
str2 = ‘I too am a string’
str3 = “”
str4 = str1 + ‘ ‘ + str2

- We can have a 0-length string as well. (str3)
- The quotes are called delimiters.
- We can combine two strings that use different delimiters. (str4)
There might arise a situation when you might need a quote inside your string. To include a delimiter in a string we enclose the string within the other kind of delimiter.
str5 = “Let’s say we need a single quote i.e. -> ‘ “
str6 = ‘What if we “need” a double quote i.e. -> “ ‘

If we need both kinds of quotes inside the string, we use escape characters. Escape characters take away the functionality of the character and render that character verbatim. In Python 3, backslash “\” is an escape character.
str7 = “Let’s escape both single quote(\’) and double quote(\”)”

Try printing the above string without the escape characters and you will see that it throws an error. Another way to escape is by using triple single-quotes (‘’’) or triple double-quotes (“””) to enclose strings.
str8 = ‘’’Yet another way of escaping single (\’) and double (\”)’’’
str9 = “””Yet another way of escaping single (\’) and double (\”)”””

These escape characters stay hidden. They are like magic cloaks. But these can also be revealed by prefacing your string with an R or r. Such a string with revealed escape characters is called a raw string.
# Raw String
str10 = R”Let’s escape both single quote(\’) and double quote(\”)”

Indexing
As mentioned earlier strings are sequences of characters. So, we can actually treat them like an array and we can access individual substrings using their positions. The first character of any non-empty string starts from index 0. To access any character at position L+1 in the string we index it by writing str[L].
# Indexing String
print(“str7[0] = “, str7[0])
print(“str7[1] = “, str7[1])
print(“str7[0:10] = “, str7[0:10])
print(“str7[10:5] = “, str7[10:5])
print(“str7[10:25] = “, str7[10:25])
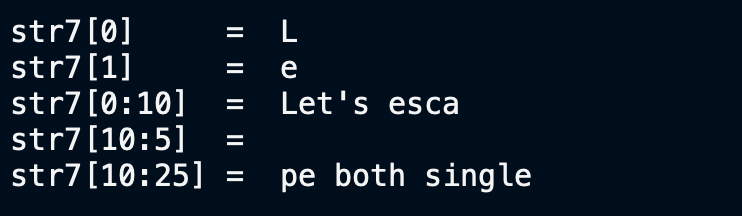
Note that even a space is counted as a character in a string.
Type Conversion
Types can be interconverted. Interconversion is useful in applications like web development where you might have to store server statistics as strings. So, you might need to convert integers and boolean values to strings.
# Type Conversion (Initial Types Used)
str1 = “15”
in1 = 10
fl1 = 10.8
Let’s try converting each type to some other type and print the results.
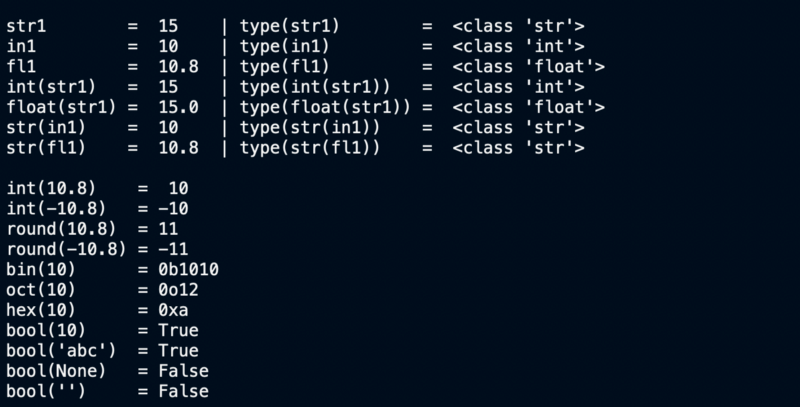
- A decimal point is added when integer is converted to a float
- Float to integer conversion also rounds down the number
- One can also change the representation of a number from decimal to binary etc.
- Bool() converts any non-empty string to True and an empty string to false.
Operators
Operators are symbols that perform an operation on one or more variables. The most common numerical operation, for example, is the addition of two or more numbers.
Numerical Operations
# Operators
num1 = 10
num2 = 4
num3 = 5e2
num1 += 1
num1 *= 3
num1 /= 2
Let’s see some operations’ outputs.
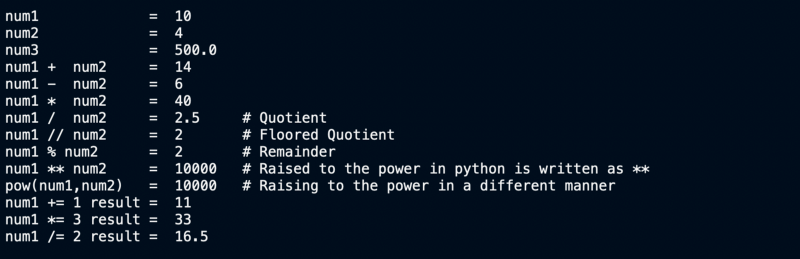
Things to note
- Note num3. It is written in a scientific notation and gets printed as a float.
- By default division (also called quotient) results in a float.
- The floored quotient rounds down the result of division.
- There are 2 ways to compute power of a number.
- Also, notice the three combined assignment statements at the bottom. These are respectively called addition assignment, multiplication assignment and division assignment operators.
Bitwise Operations
Bitwise operations make sense for integers only. Special attention must be paid to | and & operators. To clearly see them at work it is important to print your integers as binary numbers.
# Bitwise Operations
num4 = 12
num5 = 9
bool1 = True
bool2 = False
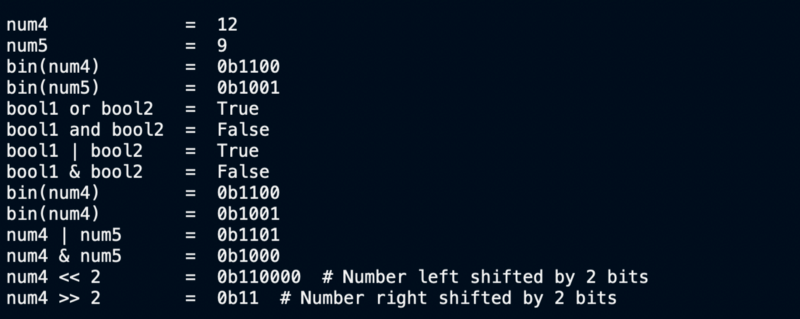
- On boolean variables or works the same as | and & works the same as and
- On numbers | operates on every bit of the number
- >> and << are used to perform bit shift operations
Data Structures
Some of Python’s built-in types also serve as data structures. Let us begin by looking at list and tuples which just like string and byte are sequence types, that is they are arranged in a sequence and can be accessed in that sequence.
List
A list can be created in one of the following ways.
- Using a pair of square brackets to denote the empty list: []
- Using square brackets, separating items with commas: [a], [a, b, c]
- Using a list comprehension: [x for x in iterable]
- Using the type constructor: list() or list(iterable)
List Indexing and slicing
Indexing means picking up a specific element at an index/position. Slicing means picking up a subset of the list.
# List Indexing
l1 = [1,2,3,4,5,6,7,8,9,10]
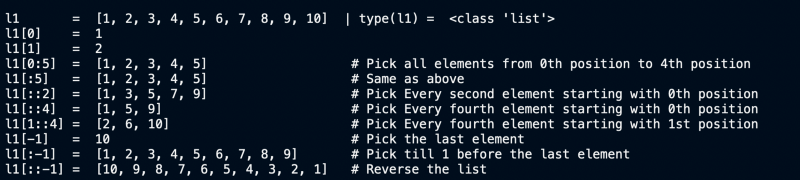
List[0:5] picks out the first 5 elements of the list. This operation is called slicing and is a peculiar feature of sequence types. Later we will see how this doesn’t apply to unordered types like sets and dictionaries.
Notice how indexing a list is similar to indexing a string. This is because in Python both of these are sequence types and are treated as an ordered data structure.
List Methods
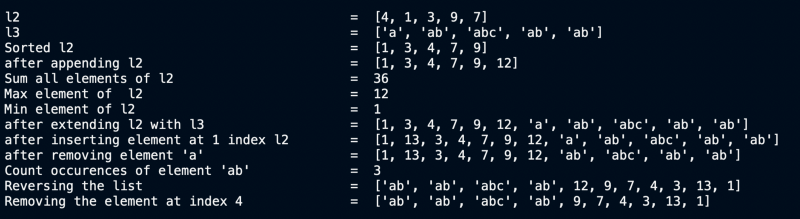
Tuple
Tuple is very similar to a list. So much so, that some people resort to calling it a cousin of the list data structure. Tuple is immutable, which means that its elements cannot be changed.
# Tuples
tp1 = (“hello”, ) # Single length tuple
tp2 = (1,2,3,[5,6]) # Tuple containing mutable types

- Tuple is enclosed in circular brackets
- A single element tuple needs a comma to distinguish it from a regular string
- We can change an element of the tuple if it is a list (or any other mutable type for that matter)
# Immutability
tp2[0] = 0

# Changing the mutable parts
tp2[3][1] = 4

But what good is a variable if it can’t be changed? In huge projects, spanning over hundred thousand lines of code and being modified by hundreds of programmers it makes sense to have a few data objects that stay constant and can’t be modified by anybody, e.g. configuration parameters etc.
Set
Set is unordered. That means it doesn’t preserve the order in which elements are added to the set. Which means that it is different from sequence types like list or string. Therefore, it doesn’t support sequence methods like indexing or slicing. A set is used to remove duplicates, and computing mathematical operations such as intersection, union and difference.
# Sets
set1 = {‘a’, ‘b’, ‘c’, ‘a’}
set2 = {‘a’, ‘d’, ‘e’, ‘f’}
set3 = {‘a’, ‘d’}

- Note that each element is unique in the set.
- The order of elements in a set is different every time it is printed.
list1 = [‘a’,’b’, ‘a’, ‘a’]
set1 = set(list1) #How to convert list to a set

- Note how a set is created from a list.
- Also, this serves as a method to find out the unique elements in a list
Dictionary
What is known as a hash in other languages is called dictionary in Python. A dictionary is a mapping type. It is also unordered but it is mutable. Dictionary is made up of key:value pairs. The keys should be hashable and all unique.
An object is hashable if it has a hash value which never changes during its lifetime, and can be compared to other objects. Hashable objects which compare equal must have the same hash value.
# Dictionary
dict1 = {‘a’: 12, ‘b’: 13, ‘c’:14, ‘d’:15}
dict2 = {‘d’ : 16, ‘e’: 17}
# Update old key and add new key
dict1.update(dict2)

Dictionaries are very useful because they allow fast searching. Secondly, most web development frameworks use APIs these days and the basic data structure in APIs is JSON which is essentially a dictionary.
Control Flow
In Python, the flow of a program can be controlled by various statements. These statements alter the flow based on certain Boolean conditions.
If/Else
If/else is the most common control flow statement. Elif is short for ‘else if’.

For Loop
For loops are used to repeat a certain block of code a predetermined number of times. Unlike C or Java the for-loop in Python loops over sequences like list, string or even a structure like dictionary as well. Let’s take a look at some examples
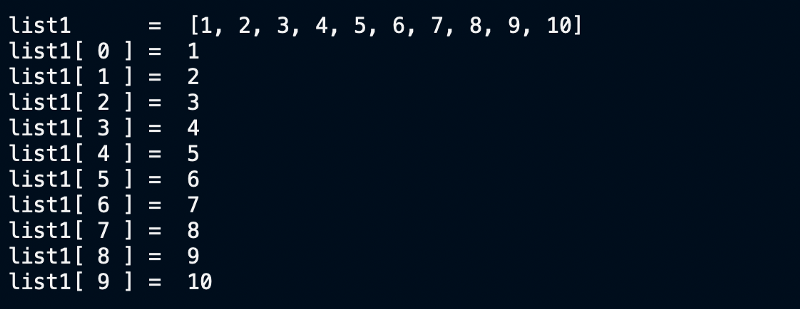
str1 = “Hello World!”
print(str1)
for c in str1:
print(c)
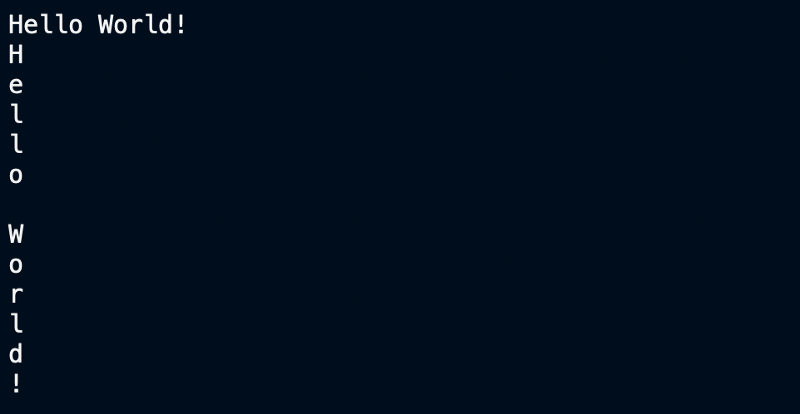
Break, Continue, Pass Statements
In case we want to break the loop upon some special condition we use the break statement. In the code block below, we will loop over a list of fruits. We know that an elephant has snuck into our fruit basket and we want to remove it. So as soon as we find elephant, we halt our loop and stop printing any more fruits.
fruits = [“apple”, “banana”, “cherry”, “dragonfruit”, “elephant”, “fig”]
print(“Available Fruits = “, fruits)
for fruit in fruits:
if(fruit == “elephant”):
print(“I cannot eat”, fruit)
break
else:
print(“I can eat”, fruit)

Continue statement is used to skip everything after itself and move on to the next iteration of the loop whereas Pass is just a filler statement.
Continue is specific to loops
In the example below see how ‘pass’ does nothing whereas ‘continue’ skips the print statement as well as the letter h
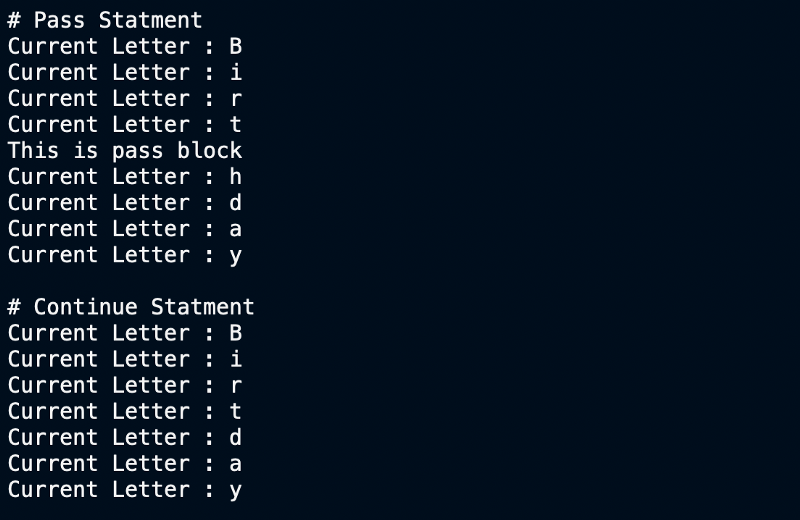
While Loop
The difference between for and while is that while keeps on going until its condition turns false.
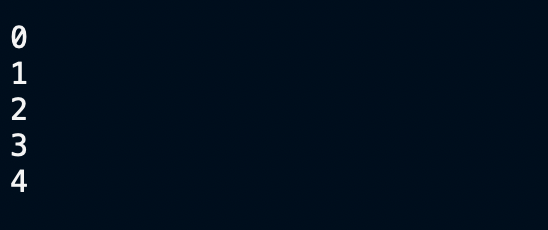
# While Loop
count = 0
while count < 5:
print(count)
count += 1
Functions
When a particular task needs to be done again and again it is wrapped inside a function. Functions which are attached to objects of a class are called instance methods. The other types of methods are the built-in methods. These are the ones that are attached to specific types e.g. len() attached to lists, dicts and sets.
Functions that we have seen till now — len(), count(), print(), sort(), append(), insert(), remove() etc. are all examples of built-in methods. A few properties of functions :
- They may accept inputs (called parameters)
- They may return information that is generated inside
Let us say we want to generate a list of numbers using while loop. The list starts at number 0 and continues till the number upper_limit. Instead of writing a while loop every time we change the upper_limit we can write a function.

- Upper_limit is a parameter of the function
- To output the generated list we use a keyword return
- Using the same function with a different parameter(argument) we could generate two different lists
Named Arguments Vs Positional Arguments
If we have multiple parameters, we need to have a way to know which argument is placed where in the parenthesis. For this we may use positional arguments or named arguments.
- Positional arguments are mentioned in the order they are defined in the function
- Named orders are defined like this argument_name = argument value and they can follow any order.

- Note how in the named arguments statement we can mention arguments in any order.
Default Arguments
Sometimes we may have some parameters that only appear occasionally. So, we provide a default value for them in the function definition in case they don’t appear.

Lambda Functions
Python allows us to create functions without a name. These functions do not contain the def keyword that we saw above. However, they contain the keyword lambda and so, they are called lambda functions. They have the following structure lambda arguments : expression
# Lambda Function
x = lambda a : a/2
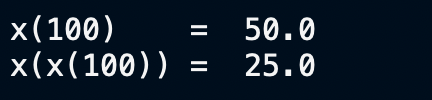
Range
Rather than being a function, range is actually an immutable sequence type like a tuple. It generates a range of numbers.

Python contains a huge list of other built-in methods. Check those out here and try some of them.
Classes
Python is an object-oriented programming language and classes are the foundation of an object-oriented design. Objects are instances of a class. Any action that is performed on these objects is done through class methods. Let’s take a look at how to create and use a class.
Creating Class and Methods
Things to Note
- Keyword class creates the class. Note that convention dictates that the class name should begin with a capital letter
- __init__ is a constructor function and it is this function which will create the object when we call Ball() function
- This class has five attributes or fields or properties named brand, age, color, game and speed.
- This class has 2 instance methods called kick and stop.
Kick method kicks the ball and gives it a random velocityStop method makes the velocity 0 or throws a warning if the ball is already not moving.
Creating Objects
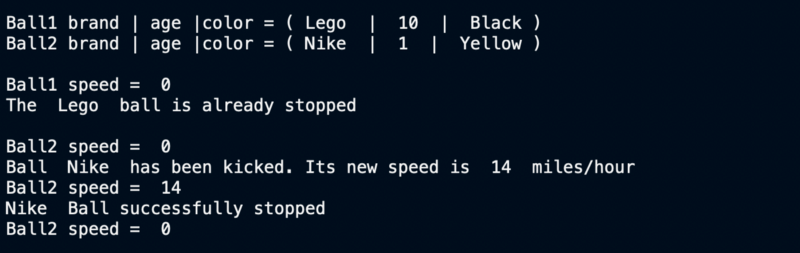
Notice that the same method is used to create both the balls. Just the arguments are different. We can access the attribute of an object by writing object.attribute e.g. Ball2.color gives us its color. We can call a method by writing object.method() e.g. Ball2.kick().The kick method modifies the speed attribute of the ball. We checked that by printing the speed separately after kicking Ball2.
File Handling
Python provides inbuilt methods to read and write files. To create and write to a text file, we call Python’s inbuilt function open. We pass the name of the file as a parameter. Another parameter dictates the mode in which the file will be opened.
“a” = Append — To append to the end of the file“w” = Write — To overwrite any existing content
“r” = Read — To read the contents
# File Writing
filename = “test.txt”
file_handle = open(filename, “w”)
file_handle.write(“Yay!! File Created”)
# File Opening
file_handle = open(filename, “r”)
print(file_handle.read())
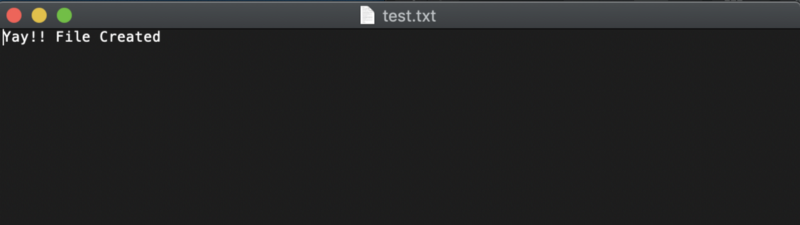
Libraries/Modules
Python is a community driven language. A library exists for every possible problem in the world. To use any external library in your program you have to import it. However, before you can import a library you need to install it using Pip. Pip is a package installer that comes bundled with Python.
PIP
To install any package, we just type
pip install package_name
So, let’s say we wanted to install a package called PyGame. It is a library to make games using Python. The following would happen once we run pip for this package.

Matplotlib
Matplotlib is a package to create data visualizations.
Look at how simple is it to import the module and start using its methods. For example, to compute sine values we imported Math module and just used math.sin(). The output plot is below.
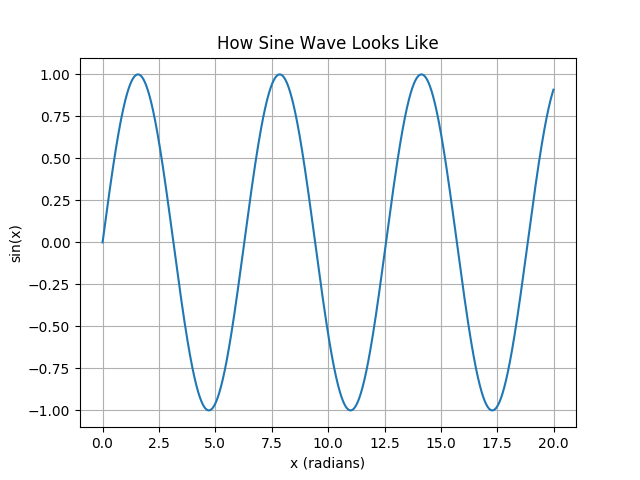
Pandas
Pandas is a key Python library for data scientists. It has a data structure called DataFrame which is like a table of data. With Pandas we can read csv/xls files and store the data in DataFrames. In the example below, we will import housing data from a csv file, store it in a dataframe and analyse that data.
Head() function shows you the first 5 rows of your data. Each row is the data of a particular household in California.
import numpy as np
import pandas as pd
house_data = pd.read_csv(“https://download.mlcc.google.com/mledu-datasets/california_housing_train.csv", sep=”,”)
print(house_data.head())

The second from the right column in the dataframe above points to medium income values for a given household. It makes sense to see how is the income distributed across all households. So we will attempt to draw a histogram of income using hist() method of dataframes.
import matplotlib.pyplot as plt
house_data[“median_income”].hist()
plt.show()
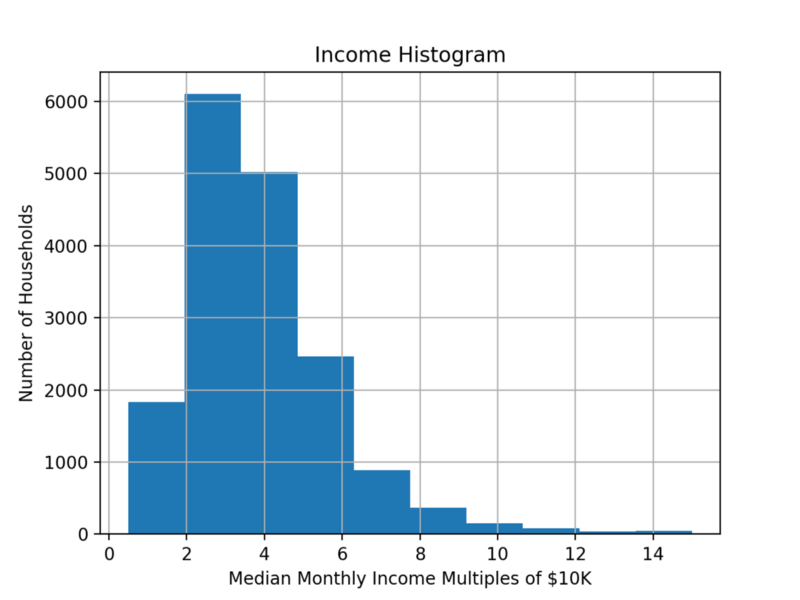
Note how the income is distributed almost in a Gaussian shape, with a few people earning above 80K and below 20K and most of them in the middle.
Beautiful Soup — The Package to Scrape Web
Beautiful soup package helps you scrape html from any webpage and break it down into its various components. For example, let’s say we want to fetch the names of all the faculty members of English Department at Cambridge University. We could write the code below.
In this code we parse the page, fetch all the hyperlinks from the html get the text from those links.
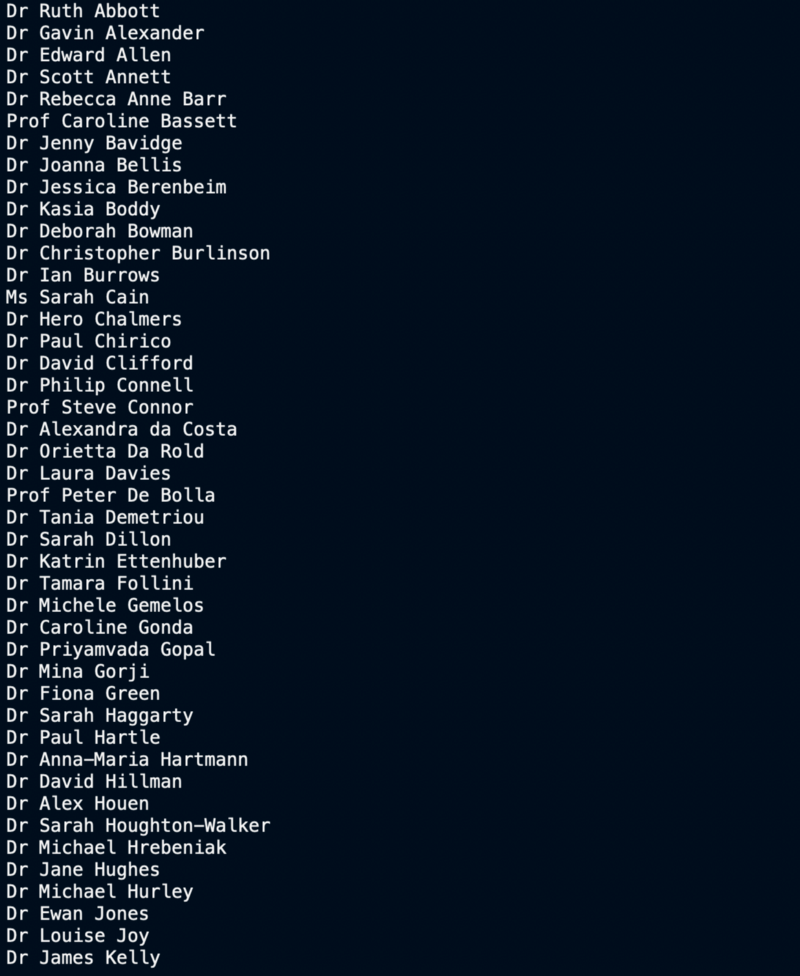
Python’s immensely rich database of packages makes it a very powerful language. I encourage you to explore other packages as well.
Jupyter
Finally, in the world of AI and Data Science Jupyter is increasingly being the go-to tool for presenting your work at conferences or meetings. You can present your Python code in a step-by-step fashion through Jupyter Notebooks. They can also be used to create and share documents with live code, equations and visualizations.
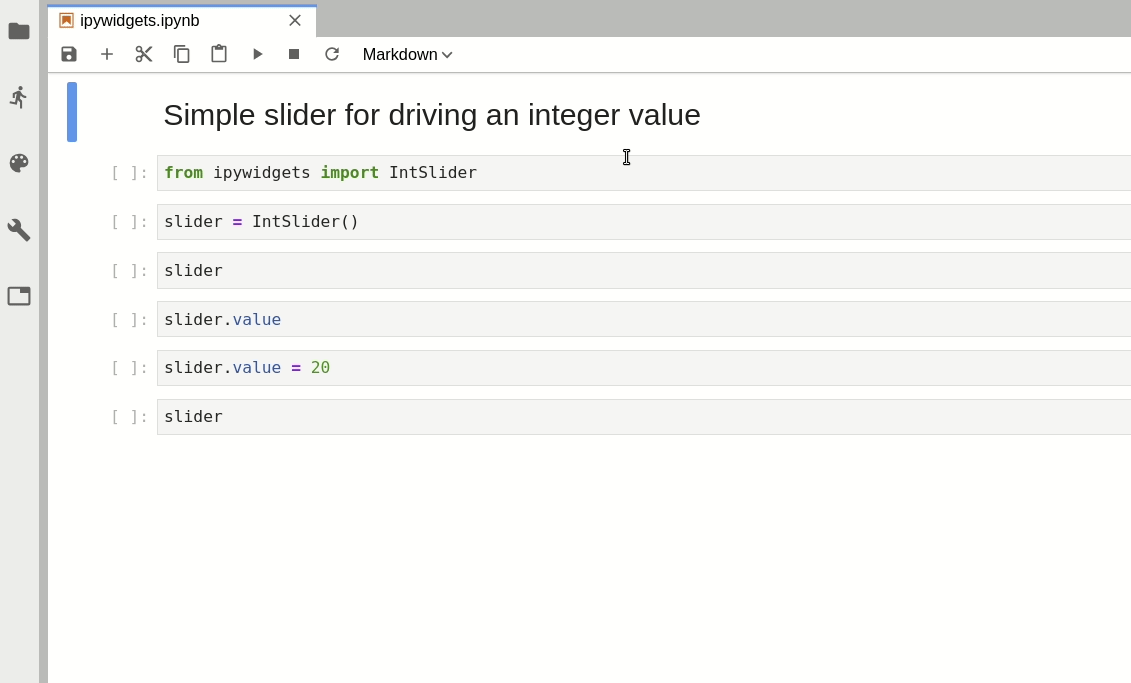
Conclusion
Python language is the Thor’s hammer in today’s world. It is easy to learn, quick to master and amazing to experiment with. It is a must have skill to be adept at Python. Just pick up any goal and start converting your coffee to code.
A different version of this cheat sheet appeared in Saturn Cloud’s blog as well. Do check them out for other informative content.
Member discussion